Meaning of ARI LEDs colors and patterns#
There are four different LEDs displays in ARI, located on the ears, the microphone (on the front of the robot) and on the back.
Ears#
Both ears have the same behaviour and the ARI’s ear LEDs have two default meanings:
yellow and red blinking ears mean that battery level is under 20%. For more details check the battery how-to;
blue and red blinking ears mean that an error on the motors occured.
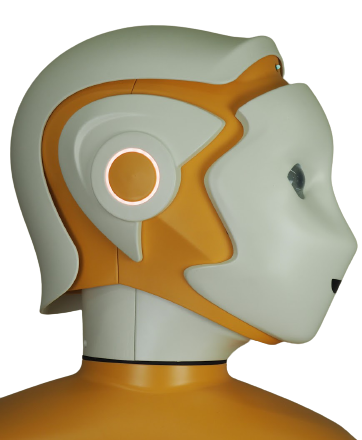
Back LEDs#
The back LEDs have three default meaning:
yellow and red blinking back LEDs mean that the batter level is under 20%. For more details check the battery how-to;
blue and red blinking back LEDs mean that an error on the motors occured;
if ARI is charging, back LEDs show the current charge level.
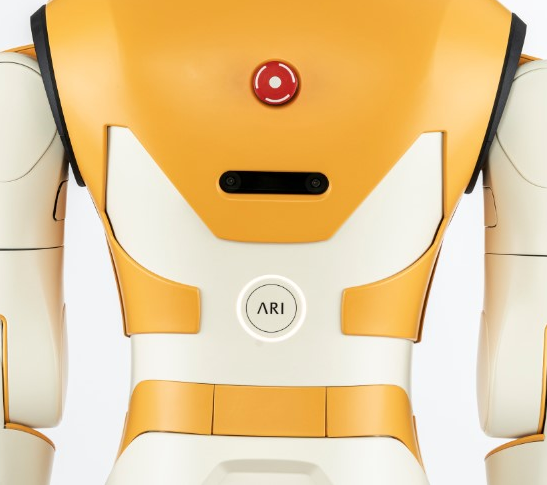
Microphone LEDs#
The microphone LEDs are blue by default.
You can change the microphone LEDs colours yourself: see Tutorial: Creating expressions with LEDs.
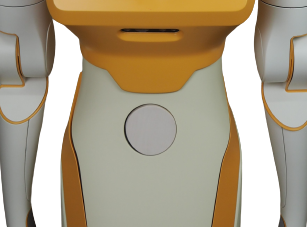
Example code#
1#!/usr/bin/env python
2# -*- coding: utf-8 -*-
3
4# System imports
5import rospy
6from actionlib import SimpleActionClient
7from pal_device_msgs.msg import DoTimedLedEffectAction, DoTimedLedEffectGoal,
8LedEffectParams
9
10
11# led devices ids
12BACK = 0
13LEFT_EAR = 1
14RIGHT_EAR = 2
15RESPEAKER = 4
16
17MAX_PRIORITY = 255 # The maximum value for leds effect priority
18
19
20def init_clients():
21 """ Initializing the action clients. """
22
23 # Creating a client object to communicate
24 # with the LEDs action server
25 led_client = SimpleActionClient("/pal_led_manager/do_effect",
26 DoTimedLedEffectAction)
27 led_client.wait_for_server()
28
29 return led_client
30
31
32def show_fixed_color(client,
33 devices,
34 r,
35 g,
36 b,
37 a,
38 duration_secs,
39 duration_nsecs=0):
40 """ Setting up and sending a LedEffectGoal
41 for a fixed color leds effect
42 """
43 goal = DoTimedLedEffectGoal()
44 goal.devices = devices
45 goal.params.effectType = LedEffectParams.FIXED_COLOR
46
47 # Setting the colour parameters
48 goal.params.fixed_color.color.r = r
49 goal.params.fixed_color.color.g = g
50 goal.params.fixed_color.color.b = b
51 goal.params.fixed_color.color.a = a
52
53 # Setting non effect-specific parameters
54 goal.effectDuration.secs = duration_secs
55 goal.effectDuration.nsecs = duration_nsecs
56 goal.priority = MAX_PRIORITY
57 client.send_goal_and_wait(goal)
58
59
60if __name__ == '__main__':
61 try:
62 rospy.init_node('led_basic_tuto')
63 led_client = init_clients()
64 show_fixed_color(led_client,
65 [BACK, LEFT_EAR, RIGHT_EAR, RESPEAKER],
66 r=1,
67 g=0,
68 b=0,
69 a=1,
70 duration_secs=10)
71 except rospy.ROSInterruptException:
72 print("Abruptly finished!")
Code explanation#
We import the required LEDs-related messages.
7from pal_device_msgs.msg import DoTimedLedEffectAction, DoTimedLedEffectGoal,
8LedEffectParams
Then, we define the different LEDs IDs.
10# led devices ids
11BACK = 0
12LEFT_EAR = 1
13RIGHT_EAR = 2
14RESPEAKER = 4
15
16MAX_PRIORITY = 255 # The maximum value for leds effect priority
Now, we are defining a set of functions that will be used later
in __main__
. The first function we implement is
init_clients()
, to initialise the client-server
connection required to interact with the ROS action server involved
in the LEDs management.
19def init_clients():
20 """ Initializing the action clients. """
21
22 # Creating a client object to communicate
23 # with the LEDs action server
24 led_client = SimpleActionClient("/pal_led_manager/do_effect",
25 DoTimedLedEffectAction)
26 led_client.wait_for_server()
27
28 return led_client
We will go through the definition of the function that initialises and sets
the fields of a DoTimedLedEffectAction
object, that is, the object
sent by the node to the action server to request a specific LED effect.
In this example, we will see how to set the Fixed Color effect.
32def show_fixed_color(client,
33 devices,
34 r,
35 g,
36 b,
37 a,
38 duration_secs,
39 duration_nsecs=0):
40 """ Setting up and sending a LedEffectGoal
41 for a fixed color leds effect
42 """
43 goal = DoTimedLedEffectGoal()
44 goal.devices = devices
45 goal.params.effectType = LedEffectParams.FIXED_COLOR
46
47 # Setting the colour parameters
48 goal.params.fixed_color.color.r = r
49 goal.params.fixed_color.color.g = g
50 goal.params.fixed_color.color.b = b
51 goal.params.fixed_color.color.a = a
52
53 # Setting non effect-specific parameters
54 goal.effectDuration.secs = duration_secs
55 goal.effectDuration.nsecs = duration_nsecs
56 goal.priority = MAX_PRIORITY
57 client.send_goal_and_wait(goal)
At this point, we have all the functions that we need to run this example. In
__main__
, we can find how we call the two functions that need it.
62if __name__ == '__main__':
63 try:
64 rospy.init_node('led_basic_tuto')
65 print("init")
66 led_client = init_clients()
67 show_fixed_color(led_client,
68 [BACK, LEFT_EAR, RIGHT_EAR, RESPEAKER],
69 r=1,
70 g=0,
71 b=0,
72 a=1,
73 duration_secs=10)
74 except rospy.ROSInterruptException:
75 print("Abruptly finished!")
See also#
check the LEDs API to know more about the available LEDs effects;
check creating expressions with LEDs for a tutorial on using LEDs to enhance expressivity.